Documentation
¶
Overview ¶
Colors and Style ¶
You can change `colors` and `styles` for some of the aspects of `gomp`
#### Let's say to you want to change Color of Artist from the default Purple to Red
In your `config.yml` ```yml COLORS:
artist:
foreground: Red
# Another Example pbar_artist:
foreground: "#ff0000" # For Hex Values bold: True # Changes the Style italic: False
```
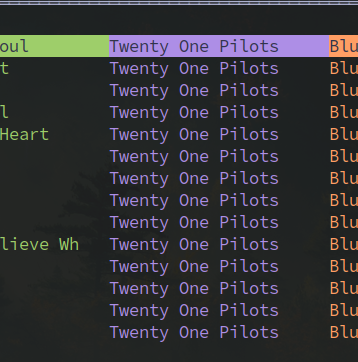 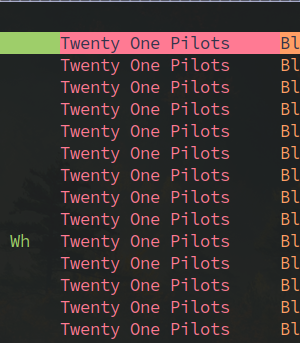
 # Configuration Configuration of gomp is done through a `config.yml` file in `$HOME/.config/gomp/`
Index ¶
Constants ¶
This section is empty.
Variables ¶
View Source
var ( ColorError = func(s string) { _s := fmt.Sprintf("Wrong Color Provided: %s", s) utils.Print("RED", _s) os.Exit(-1) } DColors = map[string]tcell.Color{ "Black": tcell.ColorBlack, "Maroon": tcell.ColorMaroon, "Green": tcell.ColorGreen, "Olive": tcell.ColorOlive, "Navy": tcell.ColorNavy, "Purple": tcell.ColorPurple, "Teal": tcell.ColorTeal, "Silver": tcell.ColorSilver, "Gray": tcell.ColorGray, "Red": tcell.ColorRed, "Lime": tcell.ColorLime, "Yellow": tcell.ColorYellow, "Blue": tcell.ColorBlue, "Fuchsia": tcell.ColorFuchsia, "Aqua": tcell.ColorAqua, "White": tcell.ColorWhite, } )
View Source
var ( ConfigPath = configDir + "/gomp" ShowVersion = false Config = NewConfigS() OnConfigChange func() )
View Source
var ( SPECIAL_KEYS = map[string]int{ "TAB": 9, "RETURN": 13, "ENTER": 13, "SPACE": 32, "[": 91, "]": 93, "(": 40, ")": 41, "{": 123, "}": 125, "<": 60, ">": 62, "?": 63, "/": 47, ";": 59, ":": 58, "'": 39, "\"": 34, } // // Following functions are provided : // // | Functions | Default Key Mapping | // |------------------------------------|---------------------| KEY_MAP = map[int]string{ 108: "showChildrenContent", 112: "togglePlayBack", 104: "showParentContent", 110: "nextSong", 99: "clearPlaylist", 78: "previousSong", 97: "addToPlaylist", 122: "toggleRandom", 114: "toggleRepeat", 45: "decreaseVolume", 61: "increaseVolume", 49: "navigateToPlaylist", 50: "navigateToFiles", 51: "navigateToSearch", 113: "quit", 115: "stop", 117: "updateDB", 100: "deleteSongFromPlaylist", 63: "FocusSearch", 47: "FocusBuffSearch", 98: "SeekBackward", 102: "SeekForward", } )
View Source
var (
VERSION = "1.0.0"
)
Functions ¶
func GenerateKeyMap ¶
func GenerateKeyMap(funcMap map[string]func())
func GetAsciiValue ¶
func ParseFlags ¶
func ParseFlags()
func ParseMPDConfig ¶
func ParseMPDConfig()
func ReadConfig ¶
func ReadConfig()
Types ¶
type Color ¶
type Colors ¶
type Colors struct { // - `album` Artist Color `mapstructure:"artist"` // - `artist` Album Color `mapstructure:"album"` // - `track` Track Color `mapstructure:"track"` // - `file` File Color `mapstructure:"file"` // - `folder` Folder Color `mapstructure:"folder"` // - `timestamp` Timestamp Color `mapstructure:"timestamp"` // - `matched_title` MatchedTitle Color `mapstructure:"matched_title"` // - `matched_folder` MatchedFolder Color `mapstructure:"matched_folder"` // - `pbar_artist` PBarArtist Color `mapstructure:"pbar_artist"` // - `pbar_track` PBarTrack Color `mapstructure:"pbar_track"` Null Color }
### Following Aspects can be changed:
type ConfigS ¶
type ConfigS struct { // ## Network Type // // By Default gomp assumes that you connect to MPD Server through tcp, But if your MPD Server is configured to expose a unix socket rather than a port, then you can specify network type to "unix" // Defaults to `tcp` if not provided. // // “`yml // NETWORK_TYPE : "unix" // “` // // Read More about it in the [sample_config](https://github.com/aditya-K2/gomp/blob/master/sample_config.yml) NetworkType string `mapstructure:"NETWORK_TYPE"` // // ## Network Address // // The Address of the Host for e.g `"localhost"` or `"/path/to/unix/socket/"` if you are using unix sockets // Defaults to `localhost` if not provided. // // “`yml // NETWORK_ADDRESS : "/home/$USER/.mpd/socket" // “` // // Read More about it in the [sample_config](https://github.com/aditya-K2/gomp/blob/master/sample_config.yml) NetworkAddress string `mapstructure:"NETWORK_ADDRESS"` // ### Default Image Path // // This is the Fallback Image that will be rendered if gomp doesn't find the embedded cover art or LastFM Cover Art. // // “`yml // DEFAULT_IMAGE_PATH : "/path/to/default/image" // “` DefaultImagePath string `mapstructure:"DEFAULT_IMAGE_PATH"` // ## Cache Directory // // By Default Images are cached to avoid re-extracting images and making redundant API Calls. The Extracted Images are copied to the `CACHE_DIR`. // // “`yml // CACHE_DIR : "/path/to/the/cache/Directory/" // “` // // Read More about Caching in the [sample_config](https://github.com/aditya-K2/gomp/blob/master/sample_config.yml) CacheDir string `mapstructure:"CACHE_DIR"` // ## Seek Offset // The amount of seconds by which the current song should seek forward or backward. // // “`yml // SEEK_OFFSET : 5 // “` SeekOffset int `mapstructure:"SEEK_OFFSET"` // ## Redraw Interval // // The amount of milliseconds after which the cover art should be redrawn if there is a event. // // “`yml // REDRAW_INTERVAL : 500 // “` RedrawInterval int `mapstructure:"REDRAW_INTERVAL"` // ## MPD Port // This is the port where your Music Player Daemon Is Running. // // ##### If not provided gomp looks for the port in mpd.conf in `~/.config/mpd/` // // “`yml // MPD_PORT : "6600" // “` Port string `mapstructure:"MPD_PORT"` // ### Music Directory // It is the path to your Music Folder that you have provided to mpd in the `mpd.conf` file. // If you do not provide the path to the `MUSIC_DIRECTORY` then gomp parses the mpd.conf file for // the `music_directory` key. // // “`yml // MUSIC_DIRECTORY : "~/Music" // “` // // The reason why you need to provide `MUSIC_DIRECTORY` is because the paths // to the songs received from mpd are relative the `MUSIC_DIRECTORY`. MusicDirectory string `mapstructure:"MUSIC_DIRECTORY"` Colors *Colors `mapstructure:"COLORS"` // ## Additional Padding // Extra padding for the placement of the image // //  // // Note: Padding can be negative or positive // `ADDITIONAL_PADDING_X` on decrementing will move the image to right // and on incrementing will move the image to left // similarly `ADDITIONAL_PADDING_Y` on decrementing will move the image UP // and on incrementing will move the image DOWN AdditionalPaddingX int `mapstructure:"ADDITIONAL_PADDING_X"` AdditionalPaddingY int `mapstructure:"ADDITIONAL_PADDING_Y"` // ## Image Width // Add extra `IMAGE_WIDTH` to the image so that it fits perfectly // in the image preview box // //  // // Note: IMAGE_WIDTH_EXTRA_X and IMAGE_WIDTH_EXTRA_Y can be positive or negative ExtraImageWidthX float64 `mapstructure:"IMAGE_WIDTH_EXTRA_X"` ExtraImageWidthY float64 `mapstructure:"IMAGE_WIDTH_EXTRA_Y"` // // # Getting Album Art from [LastFm API](https://www.last.fm/api) // // 1. Generate API Key [here](https://www.last.fm/login?next=%2Fapi%2Faccount%2Fcreate%3F_pjax%3D%2523content) // //  // // 2. Add the api key and api secret to config.yml // // “`yml // GET_COVER_ART_FROM_LAST_FM : True # Turn On Getting Album art from lastfm api GetCoverArtFromLastFm bool `mapstructure:"GET_COVER_ART_FROM_LAST_FM"` // LASTFM_API_KEY: "YOUR API KEY HERE" LastFmAPIKey string `mapstructure:"LASTFM_API_KEY"` // LASTFM_API_SECRET: "YOUR API SECRET HERE" LastFmAPISecret string `mapstructure:"LASTFM_API_SECRET"` // 3. Auto correct // //  // // “`yml // LASTFM_AUTO_CORRECT: 1 # 0 means it is turned off // “` LastFmAPIAutoCorrect int `mapstructure:"LASTFM_AUTO_CORRECT"` }
func NewConfigS ¶
func NewConfigS() *ConfigS
Click to show internal directories.
Click to hide internal directories.